Creating the "Earning Loyalty Points" Section
Step 1: Create campaigns in the Swell admin
- Log in to Swell
- Click Earning Points
- Create your Earning Points campaigns
a. This should be done after building your program strategy with your Swell Success Manager - Complete the relevant parameters for each campaign:
a. How many points should we give the customer?
b. Title: The campaign name
c. Reward text: What will be displayed to the customer as the reward
d. Description: A short description of the campaign for users to read
e. How many times each customer can complete this campaign? (relevant campaigns only)
f. If can be completed more than once What's the frequency in which the campaign can be
completed?
g. Other fields, specific to each campaign, will populate in the campaign section
Step 2: Design the rewards pages
- Design the logged-in and logged-out version of your rewards page
- In the How to earn points section, design a tile for every campaign
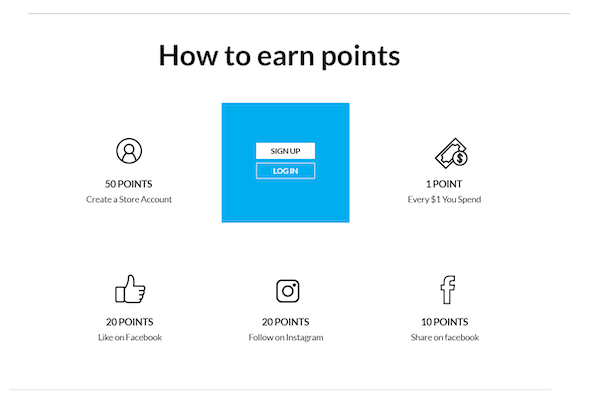
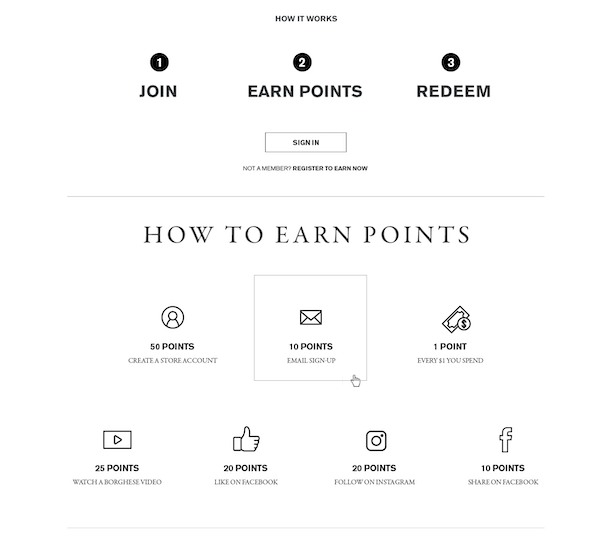
Step 3: Develop the front-end of your Loyalty pages
Before starting front-end development, install the Swell Loyalty app. Installation Guides
Refer to our Loyalty API reference to develop the Earning Points section of your loyalty program,
We recommend using the JS SDK to retrieve backend data and render it within your storefront.
1. Create campaign tiles on the front end:
Use this method to retrieve an array of campaigns. This array will hold a Campaign Object for every campaign you've set up in the Loyalty app back end.
Now that you have an array of campaigns, you can render every object as a “tile”.
On each tile, place the data from the campaign object as text.
The most commonly displayed data points used here are:
- Title
- Reward Text
- Icon / Image
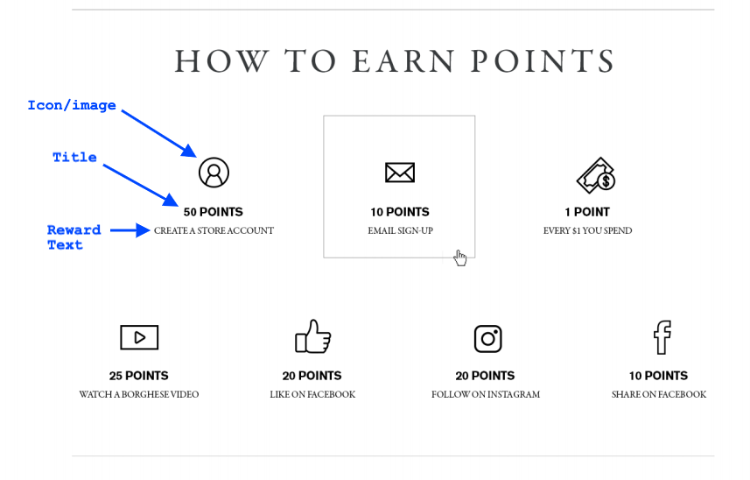
2. Creating responsive campaign tiles:
Use this markup on each tile you’ve just rendered to make the tile responsive.
The data-campaign-id
for every campaign is returned from the GetActiveCampaigns method.
We recommend using display-mode = modal to open out-of-the-box** campaign pop-ups.
When shoppers click on a tile, the tile will open a pop-up from Swell with the description of the campaign, as well as a call-to-action button if this is an interactive campaign.
Below, you'll find a sample code for creating the Loyalty API campaign section on the front end:
<section class="swell-campaign-list-container">
<h4 class="swell-campaign-list-title">Ways to earn points</h4>
<ul class="swell-campaign-list">
</ul>
</section>
<style>
.swell-campaign-list {
list-style: none;
display: flex;
width: 50%;
flex-wrap: wrap;
justify-content: center;
align-items: center;
padding: 0;
position: relative;
}
.campaign {
width: 33%;
padding: 1rem;
box-sizing: border-box;
color: black;
font-weight: 300;
border: 1px solid #eee;
position: relative;
min-height: 18rem;
display: flex;
flex-wrap: wrap;
justify-content: center;
align-items: center;
}
.campaign i {
height: 3rem;
width: 3rem;
font-size: 48px;
text-align: center;
color: black;
margin-bottom: 2rem;
}
.campaign div {
display: flex;
flex-wrap: wrap;
justify-content: center;
align-items: center;
min-height: 8rem;
}
.campaign div h5 {
width: 100%;
text-align: center;
font-weight: 300;
font-size: 12px;
}
.swell-campaign-list-title {
width: 100%;
text-align: center;
margin-bottom: 3rem;
}
button.swell-campaign-link {
padding: 0.5rem 1rem;
background-color: black;
color: white;
font-weight: 600;
width: 10rem;
border: 1px solid black;
}
li.campaign p {
width: 100%;
margin-bottom: 0;
font-weight: 800;
}
.swell-campaign-list-title {
width: 100%;
font-size: 28px;
text-align: center;
}
@media screen and (max-width: 800px) {
.campaign {
width: 50%;
}
.campaign .pink {
padding: 0.3rem 0.5rem;
}
.campaign div h5 {
width: 100%;
text-align: center;
font-weight: 300;
font-size: 12px;
}
.campaign i {
height: 2rem;
width: 2rem;
font-size: 32px;
}
.swell-campaign-list {
width: 100%;
}
.swell-campaign-list-title {
font-size: 24px;
margin-bottom: 0;
}
}
</style>
<script>
$(document).on("swell:initialized", () => {
swellAPI.getActiveCampaigns().forEach(campaign => {
$(".swell-campaign-list").append(
$("<li>").addClass("campaign").append(
$("<div>").append(
$("<i>").addClass(`fa ${campaign.icon}`),
$("<p>", {text: campaign.rewardText}),
$("<h5>", {text: campaign.title})
).attr('id', `campaign-${campaign.id}`)
).addClass("swell-campaign-link")
.attr(
{
"data-campaign-id": campaign.id,
"data-display-mode": "modal"
}
)
);
});
});
</script>
Updated about 1 month ago